Opening distribution lists
Use the MQOPEN call to open a distribution list, and use the options of the call to specify what you want to do with the list.
- A connection handle (see Putting messages on a queue for a description)
- Generic information in the Object Descriptor structure (MQOD)
- The name of each queue that you want to open, using the Object Record structure (MQOR)
- An object handle that represents your access to the distribution list
- A generic completion code
- A generic reason code
- Response Records (optional), containing a completion code and reason for each destination
Using the MQOD structure
Use the MQOD structure to identify the queues that you want to open.
To define a distribution list, you must specify MQOD_VERSION_2 in the Version
field, a value greater than zero in the RecsPresent
field, and MQOT_Q in the ObjectType
field. See MQOD for a description of all the fields of the MQOD structure.
Using the MQOR structure
Provide an MQOR structure for each destination.
The structure contains the destination queue and queue manager names. The ObjectName
and ObjectQMgrName
fields in the MQOD are not used for distribution lists. There must be one or more object records. If the ObjectQMgrName
is left blank, the local queue manager is used. See ObjectName and ObjectQMgrName for further information about these fields.
- By using the offset field
ObjectRecOffset
.In this case, the application must declare its own structure containing an MQOD structure, followed by the array of MQOR records (with as many array elements as are needed), and set
ObjectRecOffset
to the offset of the first element in the array from the start of the MQOD. Ensure that this offset is correct.Use of built-in facilities provided by the programming language is recommended, if these are available in all the environments in which the application runs. The following code illustrates this technique for the COBOL programming language:01 MY-OPEN-DATA. 02 MY-MQOD. COPY CMQODV. 02 MY-MQOR-TABLE OCCURS 100 TIMES. COPY CMQORV. MOVE LENGTH OF MY-MQOD TO MQOD-OBJECTRECOFFSET.
Alternatively, use the constant MQOD_CURRENT_LENGTH if the programming language does not support the necessary built-in facilities in all the environments concerned. The following code illustrates this technique:
However, this works correctly only if the MQOD structure and the array of MQOR records are contiguous; if the compiler inserts skip bytes between the MQOD and the MQOR array, these must be added to the value stored in01 MY-MQ-CONSTANTS. COPY CMQV. 01 MY-OPEN-DATA. 02 MY-MQOD. COPY CMQODV. 02 MY-MQOR-TABLE OCCURS 100 TIMES. COPY CMQORV. MOVE MQOD-CURRENT-LENGTH TO MQOD-OBJECTRECOFFSET.
ObjectRecOffset
.Using
ObjectRecOffset
is recommended for programming languages that do not support the pointer data type, or that implement the pointer data type in a way that is not portable to different environments (for example, the COBOL programming language). - By using the pointer field
ObjectRecPtr
.In this case, the application can declare the array of MQOR structures separately from the MQOD structure, and setObjectRecPtr
to the address of the array. The following code illustrates this technique for the C programming language:MQOD MyMqod; MQOR MyMqor[100]; MyMqod.ObjectRecPtr = MyMqor;
Using
ObjectRecPtr
is recommended for programming languages that support the pointer data type in a way that is portable to different environments (for example, the C programming language).
Whichever technique you choose, you must use one of ObjectRecOffset
and ObjectRecPtr
; the call fails with reason code MQRC_OBJECT_RECORDS_ERROR if both are zero, or both are nonzero.
Using the MQRR structure
These structures are destination-specific; each Response Record contains a CompCode
and Reason
field for each queue of a distribution list. You must use this structure to enable you to distinguish where any problems lie.
For example, if you receive a reason code of MQRC_MULTIPLE_REASONS and your distribution list contains five destination queues, you will not know which queues the problems apply to if you do not use this structure. However, if you have a completion code and reason code for each destination, you can locate the errors more easily.
See MQRR for further information about the MQRR structure.
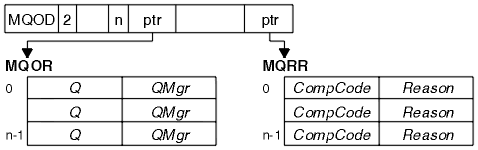

Using the MQOPEN options
- MQOO_OUTPUT
- MQOO_FAIL_IF_QUIESCING (optional)
- MQOO_ALTERNATE_USER_AUTHORITY (optional)
- MQOO_*_CONTEXT (optional)
See Opening and closing objects for a description of these options.