How to program messaging apps in JavaScript
The MQTT messaging client for JavaScript includes
a tutorial that demonstrates how to create a simple publish and subscribe
web app. By exploring the "First steps, Hello world" application code,
you can get a basic understanding of the mechanics of programming
web apps for messaging.
If your experience to date has been mainly in developing and deploying traditional messaging applications, you might also find the JavaScript coding tips section helpful. If you are an experienced JavaScript developer who is new to messaging, you'll find a brief introduction to key messaging concepts in the Messaging basics section.
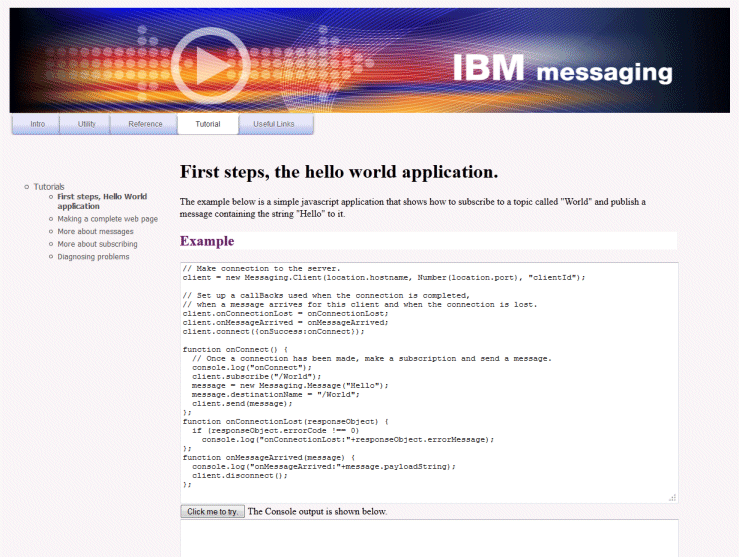
JavaScript coding tips
If
you are used to developing messaging applications, but new to web
apps, you might find the following tips helpful:
- Wrapping the code for each event in an onSuccess callback
- When you code a messaging application, you code the following
events in the following order:
- connect
- subscribe
- publish
- receive message
- Embedding the application code within HTML mark-up
- Here is an example JavaScript page:
Messaging basics
Here is some background messaging information for web app developers who are new to messaging:
- Asynchronous and fire-and-forget messaging.
- The MQTT protocol supports assured delivery and fire-and-forget transfers. In the protocol, message delivery is asynchronous: the app passes the message to the client API, and takes no further action to ensure that the message is delivered. This is approach is known as fire-and-forget. When a response is available, it is automatically sent to the app.
- An overview of publish and subscribe messaging.
- The provider of information is called a publisher. A publisher supplies information about a subject, without needing to know anything about the applications that are interested in that information. A publisher chooses a topic, which is a container for messages on a specific subject. The publisher then generates each piece of information for that subject as a message, called a publication, and posts it to the associated topic.
- How subscriptions and topics match up.
- If you are using IBM WebSphere MQ as your MQTT server, you need to understand how IBM WebSphere MQ specifies topics. In IBM WebSphere MQ, a publisher creates a message, and publishes it with a topic string that best fits the subject of the publication. To receive publications, a subscriber creates a subscription with a pattern matching topic string to select publication topics. The queue manager delivers publications to subscribers that have subscriptions that match the publication topic, and are authorized to receive the publications.