Remote Method Invocation (RMI) Adapter
The Remote Method Invocation (RMI) adapter receives requests from remote RMI clients to start business processes.
The following table provides an overview of the RMI adapter:
Category | Description |
---|---|
System name | None |
Graphical Process Modeler (GPM) category | None |
Description | Receives requests from remote RMI clients to start business processes. You can start a business process within Sterling B2B Integrator from your remote system and get the results immediately or wait for the results. The RMI adapter also enables you to check the status of a business process. The RMI client can use the RMI adapter over the Java Remote Method Protocol (JRMP). |
Preconfigured? | No |
Requires third-party files? | No |
Platform availability | All supported Sterling B2B Integrator platforms |
Related services | No |
Application requirements | The remote client can use RMI over JRMP to access this adapter. It must have the appropriate application server jar file in its classpath (for example, weblogic.jar for WebLogic). It must also have in its classpath the jar file containing the RMI stub class. Typically, the system properties java.naming.factory.initial and java.naming.provider.url are configured to select JRMP. See your application server documentation for more information. |
Initiates business processes? | Provides the remote RMI Client with the following two options for starting a business process:
|
Invocation | This adapter starts business processes but it does not run by business processes. When starting a business process through this adapter, remote RMI clients may pass parameters to that business process as key-value pairs. |
Business process context considerations | No |
Returned status values | The RMI adapter can return one of the following five statuses to the RMI Client:
|
Restrictions | The RMI adapter can only start a business process. It can send a document or key/value pairs as inputs to the business process. Business process completion status and business process resultant documents may be retrieved by the RMI client using the RMI adapter. This adapter should never be used inside an Sterling B2B Integrator business process. |
Requirements
- For security reasons, the remote client should be in the same secured area as Sterling B2B Integrator. There is no automatic secure channel associated with RMI.
If Sterling B2B Integrator and the remote client are not in the same secured area, configure JRMP to run over SSL to provide a secure channel.
- The remote client can use RMI over JRMP to access the RMI adapter.
- The remote client must have the appropriate application server .jar file in its classpath, for example, weblogic.jar for WebLogic.
- The remote client must also have in its classpath the jar file containing the RMI stub class. Typically, the system properties java.naming.factory.initial and java.naming.provider.url are configured to select JRMP. See your application server documentation for more details. For more information about .jar files, see Application Server .jar Files.
- WebLogic and JBoss support only RMI over JRMP.
How the RMI Adapter Works
The RMI adapter works with synchronous or asynchronous requests. This section explains how the RMI adapter functions for each type of request.
Asynchronous Requests
- The RMI adapter receives a request to start a business process from an RMI client, for example, runBPDocParmNoWait(). This request contains any business process parameters and documents that the business process might need.
- The RMI adapter starts a user-defined business process, and passes any input documents and business process parameters that were received with the request.
- The RMI adapter ends the business process and returns control to the RMI client. The RMI client can later poll the RMI adapter (using the business process ID) to check the completion of the business process, and to retrieve a resulting document, if applicable.
Synchronous Requests
- The RMI adapter receives a request to start a business process from an RMI client, for example, runBPDocParmWait(). This request contains any business process parameters and documents that the business process might need.
- The RMI adapter starts a user-defined business process, and passes any input documents or business process parameters that were received with the request.
- The business process completes and returns to the RMI adapter. The RMI adapter now has the final status of the business process and any associated documents.
- The RMI adapter returns to the RMI client an object (InvokedBusinessProcessDetails) containing the business process ID, business process status, and any resulting documents.
Not all business processes have documents associated with them. If a business process has multiple documents, only the primary document is returned.
The following figure shows the RMI adapter as it communicates with the external RMI client during a synchronous request:
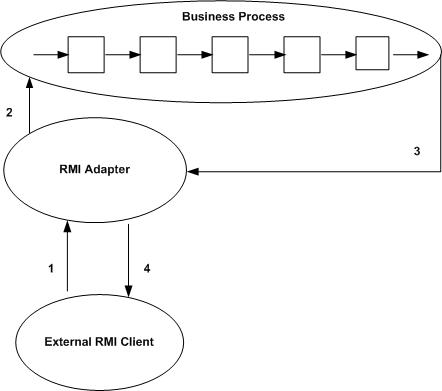
Implementing the RMI Adapter
- Create an RMI adapter configuration. For information, see Managing Services and Adapters.
- Configuring the RMI adapter. For information, see Configuring the RMI Adapter.
- Specify RMI method parameters. For information, see Starting a Business Process.
- Set system properties to make connections to the RMI adapter. For information, see Making Connections to the RMI Adapter.
- Use one of the provided RMI methods to connect to the RMI adapter and to complete a synchronous or asynchronous request. For information, see Business Process Requests.
- Use the RMI adapter in a business process.
Configuring the RMI Adapter
Because the RMI adapter is inbound and is not started by a business process, you need to configure the adapter one time only. This one configuration serves multiple clients. There are no configuration parameters other than providing a name and description for the RMI adapter.
Starting a Business Process
- Business process name – Unique name of an existing business process. Required.
- Business process document – Document to be passed to the business process. Optional.
- Name-value pairs – Name-value pairs placed at the top level of the process data for the business process being started. Optional.
- Time out value – Length of time to wait for a business process to complete. Optional.
- Security credentials – Data needed for authentication. Required.
Making Connections to the RMI Adapter
On the client Java Virtual Machine (JVM), set the following system properties for WebLogic RMI over JRMP:
java.naming.factory.initial=org.jnp.interfaces.NamingContextFactory
java.naming.provider.url=jnp://100.100.100.100:1000
java.naming.factory.url.pkgs=org.jboss.naming:org.jnp.interfaces
The following code retrieves the interface for starting the RMI adapter methods:
//Get an Initial Naming Context
Context initialNamingContext = new InitialContext();
//Find the RMI Adapter in the JNDI tree
Object obj=initialNamingContext.lookup("IIOPBusinessProcessProxy");
//Narrow the retrieved object to the interface for Invoking Business Processes
IBusinessProcessProxy proxy=(IBusinessProcessProxy)
PortableRemoteObject.narrow(obj,IBusinessProcessProxy.class);
Business Process Requests
The RMI adapter provides several RMI methods that the remote RMI client can use to start business processes and pass documents and business process parameters to those business processes.
- Invoke Business Process and Wait – The runBPDocWait() method call (synchronous request) runs on the server (or adapter). The call will not complete until the business process completes.
RMIIOPAdapterDocument doc=new RMIIOPAdapterDocument(); //Set up document to pass to business process doc.setName(document name); doc.setSubject(document subject); doc.setContentType(document content type); doc.setContentSubType(document content subtype); doc.setBody("documentbodyishere".getBytes()); //Invoke the business process, giving it an initial document and wait for completion. It will wait for the default timeout period. See BusinessProcessProxy for the default timeout period. InvokedBusinessProcessDetails bpDetails=null; try { bpDetails=proxy.runBPDocWait(NameOfTheBPToInvoke, doc, securityCredentials); } catch(Exception e) { //If a Timeout occurs then a BusinessProcessException is returned with TIMEOUT_EXCEEDED. System.out.printIn("Security, Remote, or BusinessProcess exception has occurred"); System.out.exit(-l); } //Get the returned document String returnedDocument = null; if(bpDetails.getBody()!=null) { returnedDocument=new String(bpDetails.getBody()); }
- Invoke Business Process and Return Immediately – The method call, runBPNoWait (asynchronous request), starts the business process and immediately returns the business process ID. It does not wait for the business process to complete. The user can request the status of the business process at a later time, using the getBPStatus() and getBPDocument() methods on the server and supplying the business process ID.
String bpID=null; try { bpID=proxy.runBPNoWait(NameOfTheBPToInvoke,securityCredentials); } catch(Exception e){ System.out.printIn("Security, Remote or BusinessProcess exception has occurred"); System.out.exit(-l); }
- Request Invoked Business Process Status – The getBPStatus() method retrieves the status of a business process that was started earlier. You must supply the business process ID. Note that a business process could run for a long time. Account for this when writing your client code.
//Get the current status of the invoked business process. String status=null; try { status=proxy.getBusinessProcessStatus(bpID, securityCredentials); } catch(Exception e) { System.out.printIn("Security, Remote or BusinessProcess exception has occurred"); System.out.exit(-l); }
- Retrieve Resultant Document of the Invoked Business Process – The getBPDocument() method retrieves the resultant document from the business process. If the business process completes successfully, the resultant document is returned to the user as an RMIIOPAdapterDocument object; otherwise, null is returned.
RMIIOPAdapterDocument aDoc=null; try { aDoc=proxy.getBusinessProcessDocument(bpID, securityCredentials); } catch(Exception e) { System.out.printIn("Security, Remote exception has occurred"); System.out.exit(-l); } if(aDoc==null) { //Business Process must not be complete or BP ID is invalid, etc.. } else { //get the returned document as a string String document = new String(aDoc.getBody()); }
Developing Client Code
Note the following specifications when creating code for the RMI external client method calls.
RMI Adapter .jar Files
The RMI adapter has a .jar file containing adapter class files for developing client code. This .jar file needs to be in the RMI client classpath.
Application Server .jar Files
Each application server has a supporting .jar file or files. Include these files in the client classpath. Each application server packages its .jar files differently. Therefore, the RMI client developer must locate the appropriate .jar file to support an RMI client application.