Building and deploying Spring Boot applications
You can build your Spring Boot applications for use in CICS with Maven or with Gradle.
Building Spring Boot applications as WAR files
You can build Spring Boot applications as a web application archive (WAR), which allows you to integrate Spring Framework transactional management or Spring Boot Security in CICS. See Table 1. When built as a WAR, a Spring Boot application can be deployed and managed by using CICS bundles in the same way as other CICS Liberty applications.
Capability | Spring Boot applications built into: |
---|---|
WAR | |
CICS JCICS API | Yes |
Java Persistance API (JPA) | Yes |
Spring security integration with CICS | Yes |
Spring transaction integration with CICS | Yes |
Java Database Connectivity (JDBC) | Yes |
Threading and concurrency | Yes |
Java Message Service (JMS) | Yes |
The following diagram displays the different options that you can take to run your Spring Boot application on CICS.
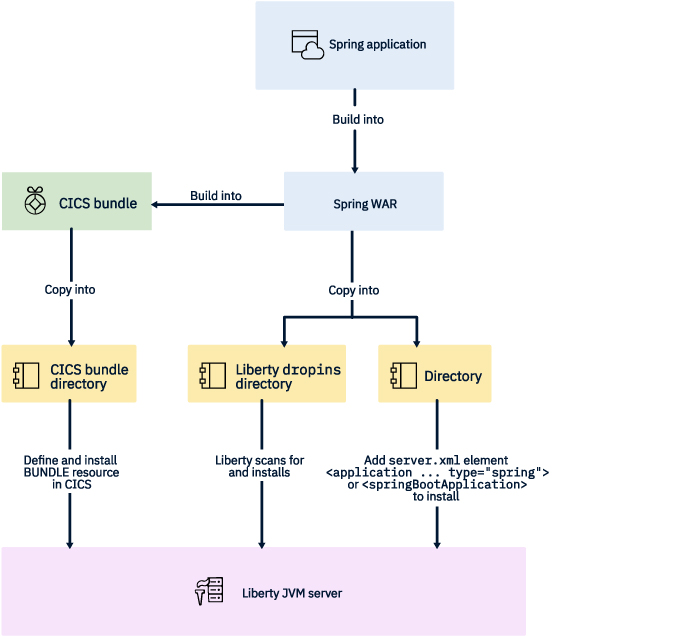
@SpringBootApplication
public class MyApplication extends SpringBootServletInitializer
{
@Override
protected SpringApplicationBuilder configure(SpringApplicationBuilder application)
{
return application.sources(MyApplication.class);
}
public static void main(String[] args)
{
SpringApplication.run(MyApplication.class, args);
}
}
For detailed information about creating a deployable WAR file with Maven or Gradle, see Create a deployable WAR file in the Spring Boot documentation.
For more information about building applications with Maven and Gradle, see Developing applications using Maven or Gradle